Hacker Typer
This challenge contained a link to a website where I had to type and submit the words that appeared on the screen as quickly as possible.
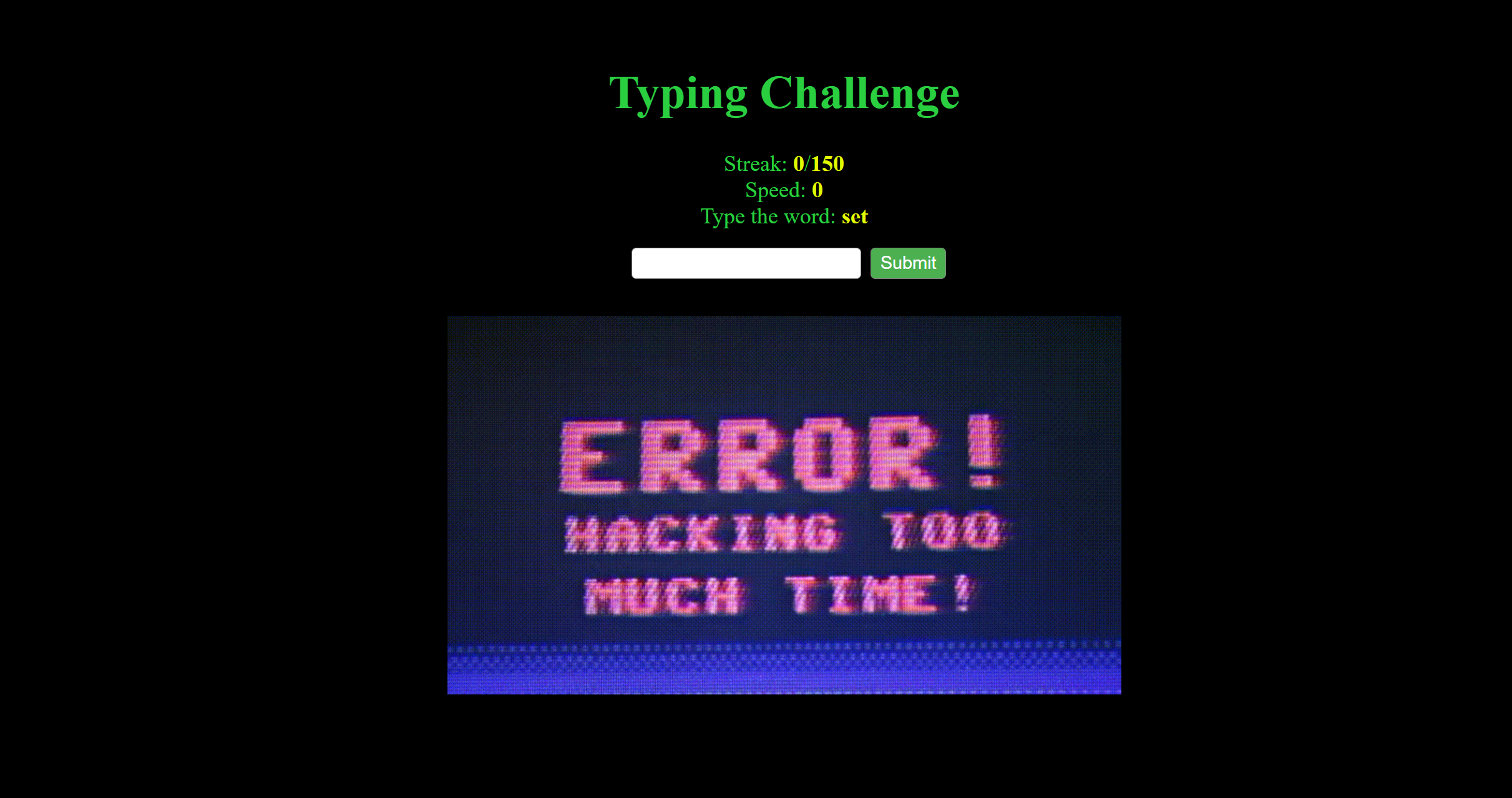
I quickly realized that typing by hand is not fast enough, so I needed to use an automation to do it quickly in order to solve the challenge.
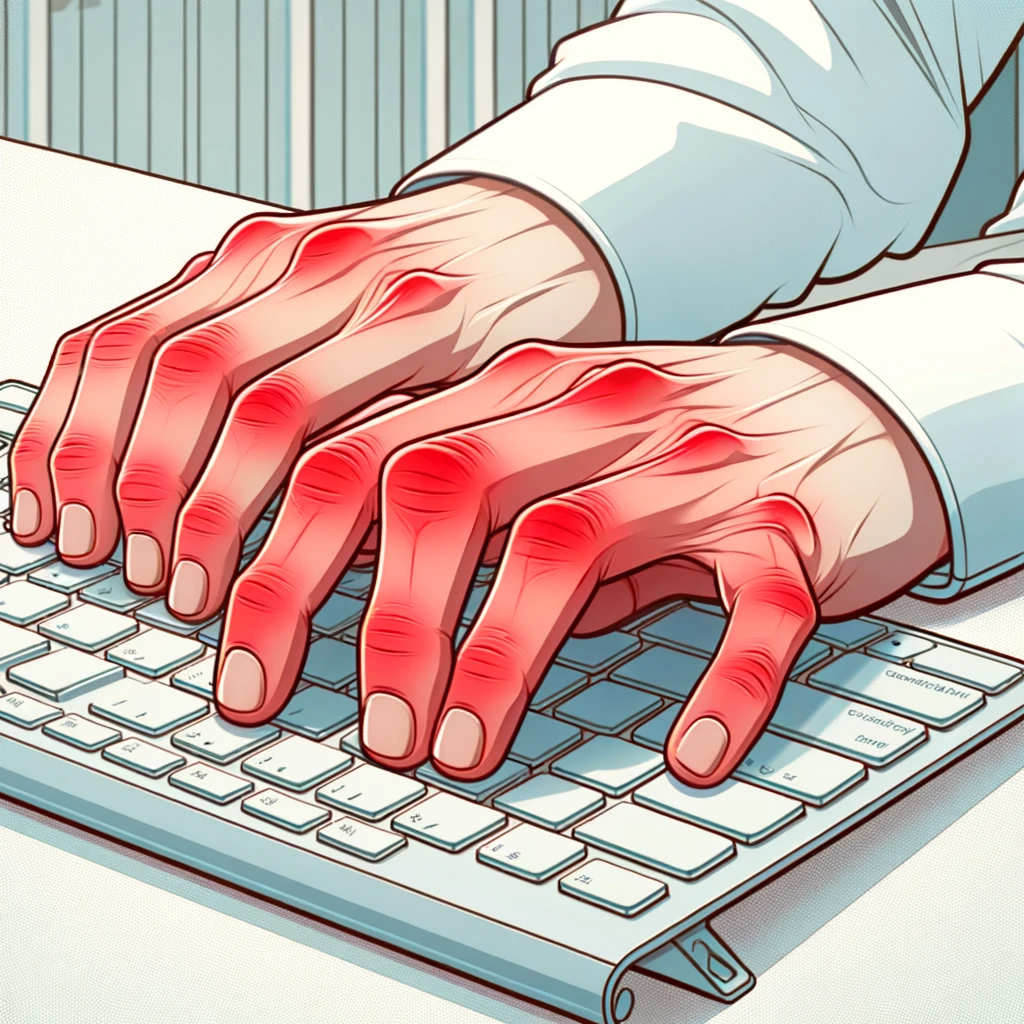
I learned some basics of Web Automation using Python and Selenium.
Selenium is used in Web Automation for automating browsers to perform tasks such as testing and web scraping.
A critical component of this process is the use of WebDriver, which is important for controlling browsers. For example, ChromeDriver is used specifically for Google Chrome.
I have created the following python script which uses Selenium to interact with a the web page. It opens the URL, reads a word from the page, inputs this word into a form, and submits it.
This process repeats continuously within a loop, with pauses to allow for page loading and response.
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
# URL of the website
url = 'https://hacker-typer.tuctf.com/'
# Set up the Selenium WebDriver (example using Chrome)
driver = webdriver.Chrome()
# Open the website
driver.get(url)
# Allow some time for the page to load
time.sleep(2)
# Loop to interact with the game
while True:
try:
# Find the element containing the word by its name attribute
word_element = driver.find_element(by='name', value='word-title')
word = word_element.text
# Find the input field and submit button
input_field = driver.find_element(by='name', value='word')
submit_button = driver.find_element(by='css selector', value='button[type="submit"]')
# Enter the word and submit
input_field.send_keys(word)
submit_button.click()
# Wait for the response and next word
time.sleep(1)
# Add any conditions to break the loop if needed
except Exception as e:
print(f"An error occurred: {e}")
break
# Close the WebDriver when done
driver.quit()
I ran the Python script.
All that remains is to wait a few minutes for the script to finish and obtain the flag.
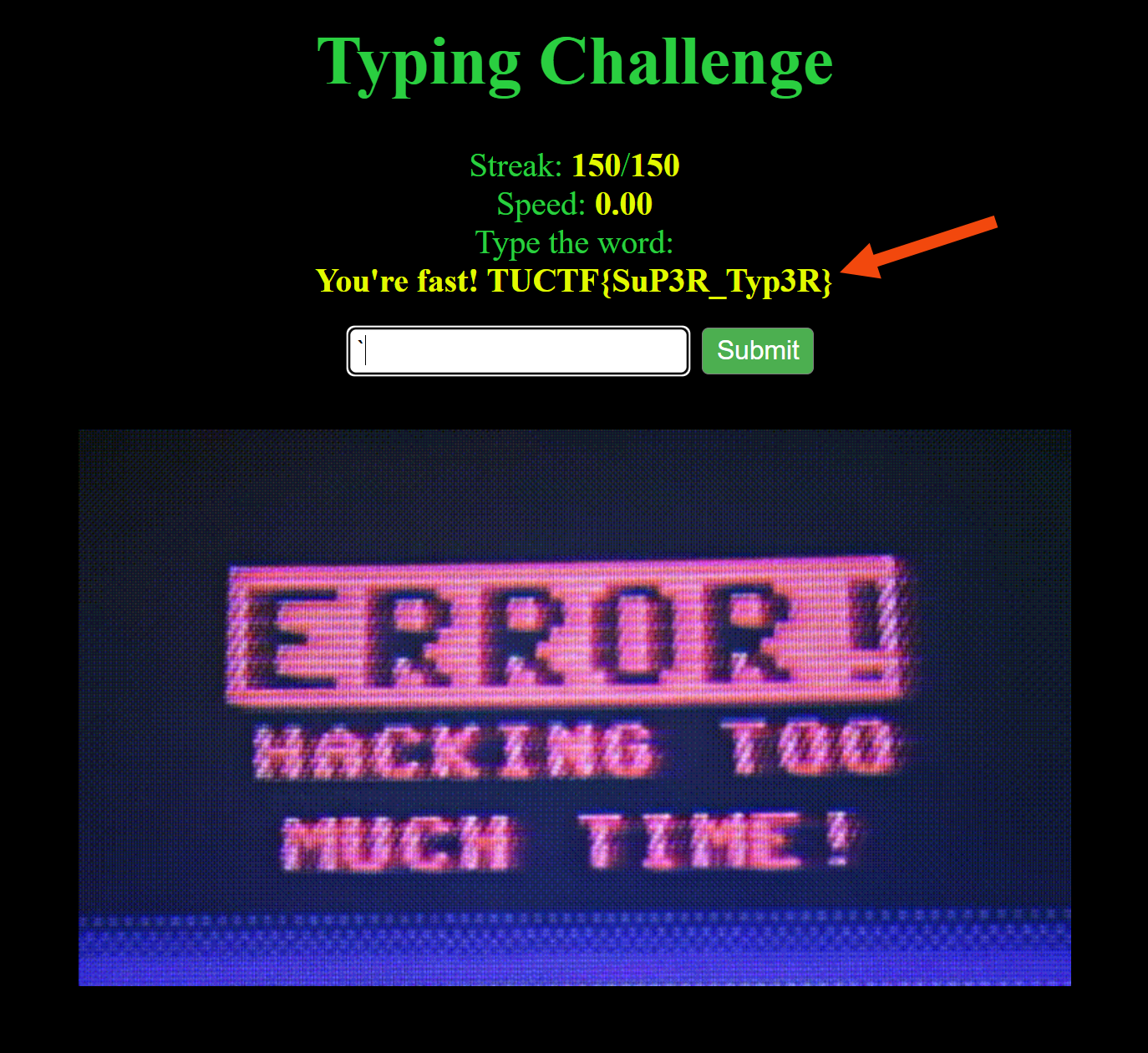
Flag: TUCTF{SuP3R_Typ3R}