Bludgeon the Booty
The challenge provided the following netcat command: nc chal.tuctf.com 30002
.
After using netcat, I needed to crack the treasure chest's code to get the flag.
Here is an example of how it looked like:
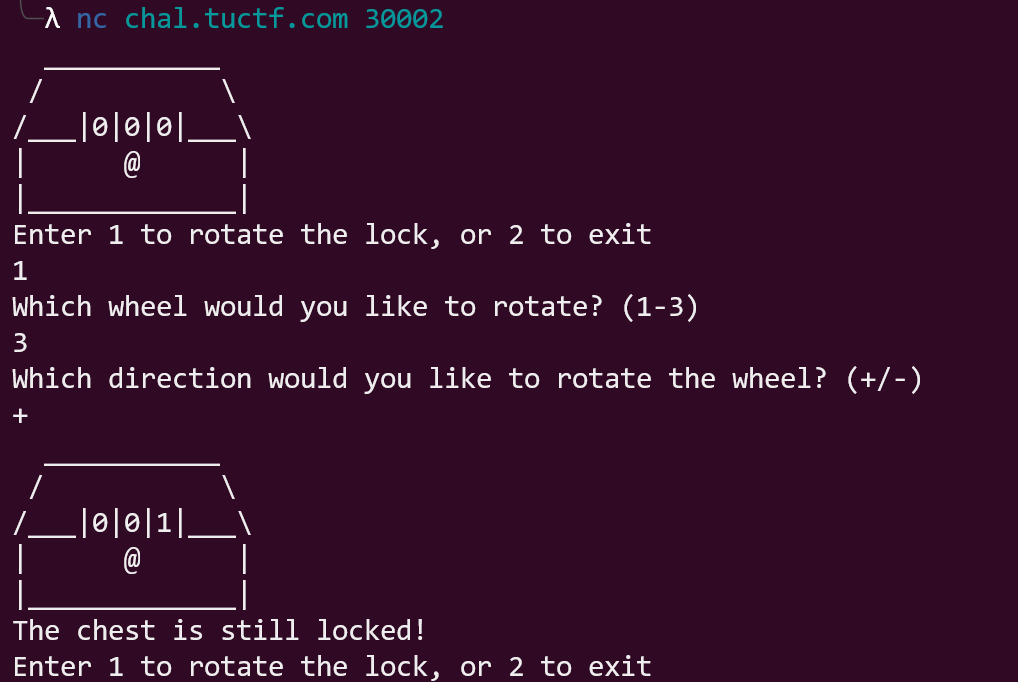
I wanted to create an automated script that could execute these steps in order to guess the code that unlocks the treasure chest.
The following Python connects to "chal.tuctf.com" on port 30002. It performs the following steps:
- Establishes a socket connection with the server.
- Receives and prints the initial message from the server and appends it to a file.
- Enters a loop where it sends the number '1' to the server to initiate a rotation of the lock.
- Receives and prints the server's response after sending '1'.
- Generates a random number between 1 and 4 and sends it to the server.
- Receives and prints the server's response after sending the random number.
- Sleeps for 2 seconds.
- Sends a '+' character to the server.
- Receives and prints the server's response after sending '+'.
The script continuously repeats steps 4 to 9 within the loop. It also logs the communication between the client and server in a file located at "C:\Users\user\ctf\TUCTF\output.txt."
import socket
import time
import random
# Define the server address and port
server_address = ("chal.tuctf.com", 30002)
file_path = r'C:\Users\user\ctf\TUCTF\output.txt'
# Create a socket object
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
# Connect to the server
client_socket.connect(server_address)
# Wait for the initial prompt from the server
initial_message = client_socket.recv(1024).decode()
print(initial_message)
with open(file_path, "a") as file:
file.write(initial_message)
# Wait for the expected prompt from the server
# while not initial_message.endswith("exit"):
# initial_message = client_socket.recv(1024).decode()
# Send '1' to the server
for i in range(9999):
client_socket.send("1\n".encode())
initial_message = client_socket.recv(1024).decode()
print(initial_message)
with open(file_path, "a") as file:
file.write("1")
with open(file_path, "a") as file:
file.write(initial_message)
number = random.randint(1, 4)
with open(file_path, "a") as file:
file.write(str(number))
client_socket.send(f"{number}\n".encode())
initial_message = client_socket.recv(1024).decode()
print(initial_message)
with open(file_path, "a") as file:
file.write(initial_message)
time.sleep(2)
client_socket.send("+\n".encode())
initial_message = client_socket.recv(1024).decode()
print(initial_message)
with open(file_path, "a") as file:
file.write("+")
with open(file_path, "a") as file:
file.write(initial_message)
# Receive and print the server's response
except Exception as e:
print(f"An error occurred: {e}")
finally:
# Close the socket
client_socket.close()
I ran the script:

All that remains is to wait for the script to finish and get the flag.
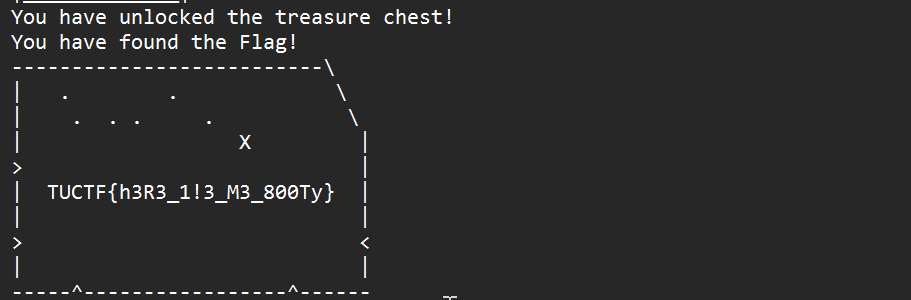
Flag: TUCTF{h3R3_1!3_M3_800Ty}